Bringing the client closer to their product using Contentful
Chloé Caron8 min read
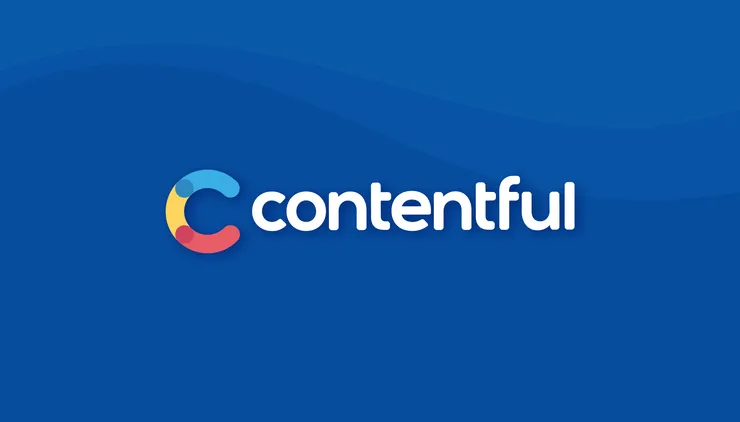
You need to make sure that your website content keeps up-to-date with changing designs and client requirements. The trade-off? This often impacts developer time. Small changes, like adjusting an image or a piece of text, seem like a 10-minute job. In reality, they take up more capacity as they still need to go through the full development processes (at Theodo these include code review, deploying, functional review, validation, etc. to ensure quality and avoid regressions).
Content Management Systems (CMS)
Utilising a CMS means that you can create, manage and modify the content of a website without needing any code changes. A developer has to integrate the CMS using its API, however, once this is done, anyone with the correct permissions can adjust the content. This means that content changes now become much simpler and the client can benefit from up-to-date content, as well as saving developer time over the longer term.
Introducing Contentful
Contentful goes beyond CMS; not only does it allow you to plug adjustable content into your website, it also incorporates additional features to optimise your content.
As a quick introduction, Contentful is a content management platform with a very comprehensible Content Delivery API. It is available in the most popular programming languages (e.g. Javascript, Python), a great help when you are working on a variety of projects utilising different stacks. Overall, some of the best advantages include the ease of setup, clear and practical JavaScript SDK, and Contentful’s intuitive platform.
Before delving into the advantages and disadvantages of Contentful, let’s introduce the technical side: how to integrate it into your code.
Code example
Setting up
The first, and essential, part of using Contentful is to create an account on their platform https://www.contentful.com/. Assuming that this has all been done, we can now turn our eye to integrating it with our code.
If you don’t yet have Contentful installed and are using Node.js, you just need to install the package using:
npm install contentful
Next, you need to initialise the client. To do this, you will need an API key and space ID (some quick steps can be found here). Although optional, you can also specify an environment. We’ll talk about this later, but it is another key advantage of using Contentful.
var client = contentful.createClient({
space: '<space_id>',
accessToken: '<access_token>',
environment: '<environment_name>',
});
That’s all you need! Once Contentful has been set up, you can now use it throughout your project.
Fetching content
There are lots of ways of retrieving content.
You can use a specific entry id to retrieve a specific item, however, you can also retrieve groups of content.
One of the most useful tools to retrieve content is being able to retrieve by content type.
Within the Contentful platform, you can create ‘content types’ that define the types of data that you will be adding to your website.
For example, you might have a simple type such as colour
which will just have a short piece of text (i.e. a title) or you might have a more complicated type such as author
which will require a name, a description, an image, etc.
To fetch this content type (e.g. fetch all the author
types), you need to call:
const authorResponse = await client.getEntries<Author>({
content_type: 'author',
})
Where Author
, in this case, is the type defined by Contentful which needs to be imported.
The response can then be used anywhere you need.
Within Typescript, the Author
type would need to be defined.
Although this can be done manually, it is more efficient to use an automatic type generator such as this one.
Optimisation
Contentful can also be used to adjust and optimise your content. A key example is with images. There are many options available (see documentation), however one of the most interesting ones I came across was the ability to change the quality of the image. By reducing the quality of the image, the content can be rendered more quickly on your page, avoiding delays. Once you have retrieved the image URL from Contentful, all you need is to adjust it and specify the quality:
const imageUrlWithAdjustedQuality = `${imageUrl}?fm=jpg&q=${quality}`
With Contentful, the quality is a percentage and can be set to be between 1 and 100.
These are just two examples of what you can do with Contentful. I would highly recommend exploring their documentation and having a look at implementing it yourself too.
Environments
Earlier, I mentioned that we can set the environment when initialising the client.
Contentful has a wonderful feature where you can have content in different environments.
This means that changes made in Contentful don’t need to directly impact your production website.
Contentful does this in a very similar way to Github where you can have different branches (e.g. master
, staging
), create and destroy branches.
The key process to releasing a new branch is as follows:
- Create a new branch (copied off of the master branch) where you make all your content changes
- Once the changes are complete, point
master
at this new branch - You can either delete the old
master
branch or you can keep it in order to revert to an older version if needed
Although this can be done through the terminal, it is highly recommended to use the Contentful platform as it is quite straightforward and can avoid unwelcome surprises.
Key advantages of using Contentful
A key feature that singles out Contentful compared to other CMS is the ability to manipulate images. It does so by not only adjusting the format and size of your image, but also by enabling cropping and adjusting the quality you would like to have - a great optimiser of loading speed.
While CMS is often thought of as being able to integrate content such as images, videos and text, Contentful takes it a step further. While the general page layout is predetermined, its content can be completely set through Contentful. This is because text can be done in markdown, giving more flexibility to what can be displayed.
In the code example, we had a look at fetching all items within a content type.
If all the data is useful to you, then this is great.
However, you may only want to fetch a specific group of items.
For example, if you have a Book
content type, you may only want to fetch items with the field genre
corresponding to 'Mystery'
.
This is done using relational queries, e.g. using the API call:
https://cdn.contentful.com/spaces/my-space-id/entries/
?content_type=books
&fields.genre.sys.contentType.sys.id=genre
&fields.genre.fields.label[all]=Mystery
However, the key benefit of using Contentful compared to other CMS is the simplicity of integration.
Disadvantages and a word of warning
Speed
Fetching all your content from Contentful seems like a dream come true, no more hardcoding text and images, freeing up developer time and making sure that your content is up-to-date. However, this does come with a warning. Fetching all the content whenever a page is loaded can be very slow. The more data you fetch from Contentful, the longer your loading time. However, this does not necessarily need to be an issue depending on which framework you are using. Contentful used alongside NextJS is one of the best solutions. NextJS uses static site generation (SSG), meaning that your content does not need to be refetched whenever you load a page - a significant time-saver. However, this does mean that if you make changes to Contentful, you will need to rebuild the site in order to see these changes. Rather than SSG, you can also look into using Incremental Static Regeneration (ISR) which uses static-generation per page rather than the entire site and would avoid the need to rebuild the entire site when a change is made on Contentful.
Field type
When fetching content types, there are also limitations on the field type we can filter on.
One of the types that it is not possible to filter by is Array<Link>
.
A further note to consider about the Link
type is the issue of depth.
Links are a great way to model the relationship between content items, however as the depth increases, this can cascade into a circular relationship.
By default, you will only access the top level, however, if you do need a higher depth, it is important to understand the possible consequences of expanding the maximum depth.
Other points to consider
Another point to consider is that Contentful cannot be installed locally for local development. To work on your project with your content fetched from Contentful, you will need to have an internet connection. However, as this is also the case for other CMS, it is not a strong disadvantage. One of its key challenges is its cost. At about $489/month for a team, Contentful can be quite costly, especially for smaller projects.
Conclusion
Overall, Contentful is a great way to optimise time for developers and ensure that the client has control of their content. However, you have to be careful about when to use this as projects with a low budget and with small amounts of content might not need such a powerful tool. Another key consideration is whether you are using NextJS on your project. If you are not, you might want to think about switching over to make sure that your content is loaded quickly and efficiently.
More documentation: https://www.contentful.com/developers/docs/references/content-delivery-api/