Setup VS Code for Efficient PHP development š
Louis-Marie Michelin9 min read
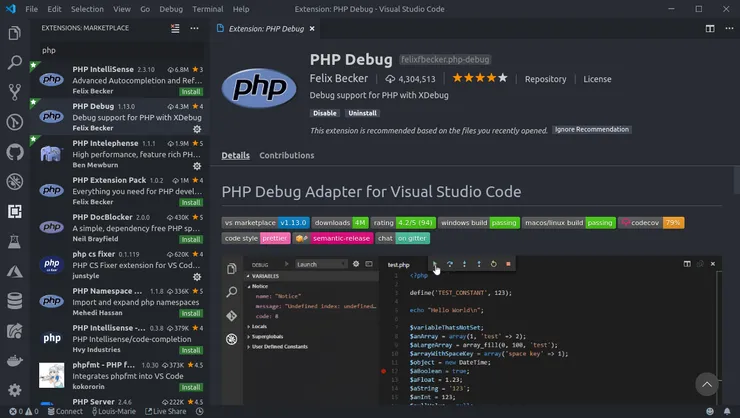
I recently started programming in PHP using the well-known Symfony framework. I wanted to keep my VS Code habits and proficiency I had from my previous projects in Node.js and Vue.js, so I tried to configure VS Code for PHP development as well. Moreover, I didnāt want to invest ā¬199 in the famous PHPStorm IDE⦠š
TL;DR
VS Code extensions you should install and configure for PHP development:
In this article, I will explain how I managed to make my development environment comparable to PhpStormās. The commands will be detailed for Ubuntu 18.04, but they can be adapted for Mac OS and possibly for Windows.
I will take an empty Symfony application as an example for this article. Letās start!
Prerequisites
Install PHP and Composer
To be able to run PHP code you obviously need to install PHP. You will also probably need Composer, a commonly used dependency manager for PHP. You can install them by running the following command:
sudo apt install php-cli composer
You can install php-all-dev
instead of php-cli
if you want to install some useful libraries as well.
Create an empty Symfony project
Letās now create an empty Symfony app. Then open VS Code and launch the development server:
composer create-project symfony/website-skeleton my-symfony-website
cd my-symfony-website
code .
bin/console server:run
Increase the number of system watchers if needed
VS Code needs to watch for file changes to work correctly. Thatās why the following warning may appear because our Symfony project contains a lot of files:
To fix it, you can run the following commands in your terminal, and then restart VS Code:
echo fs.inotify.max_user_watches=524288 | sudo tee -a /etc/sysctl.conf
sudo sysctl -p
Go toĀ http://127.0.0.1:8000Ā from your favorite web browser and check that your Symfony website works.
Configure VS Code for PHP
Autocomplete & Intellisense & Go to definition
Letās create a new route in our application. Just add an empty fileĀ HelloWorldController.php
Ā in theĀ src/Controller
Ā folder.
Install PHP Intelephense
By default, VS Code provides some basic code suggestions but they are generic and quite useless. To get useful code suggestions as you type, I recommend installing the PHP Intelephense extension for VS Code. I also tried theĀ PHP Intellisense extensionĀ but itās slower on big projects and provides fewer features (it doesnāt gray out unused imports for example).
Now that theĀ PHP Intelephense extensionĀ is installed, we can start writing our HelloWorldController!
As you can see, code suggestions are very relevant and VS Code auto imports the corresponding namespace when you validate a suggestion!
Disable basic suggestions
PHP Intelephense can also auto-suggest relevant methods when typingĀ $myVar->
. The problem is that these suggestions are polluted by the default PHP suggestions.
Basic PHP code suggestions we want to disable
To fix that, you can disable these basic suggestions in VS Code settings. Simply open VS CodeāsĀ settings.json
Ā file (pressĀ Ctrl + ,
Ā then click on the top rightĀ {}
Ā icon) and add the following line:
"php.suggest.basic": false,
When itās done, you can see useful suggestions!
Relevant suggestions when basic suggestions are disabled
Enable autocomplete in comments / annotations
When you write PHP code, a good practice is to add PHPDoc annotations to make your code more understandable and to help your IDE provide you with relevant code suggestions. By default, VS Code doesnāt suggest anything while writing annotations. To activate code suggestions in comments, open theĀ settings.json
Ā file and add the following line:
"editor.quickSuggestions": { "comments": true },
Code suggestions in annotations
Allow autocomplete in tests
By default, PHP Intelephense excludes Symfony test folders from indexing because the default exclude settings contains a too generic pattern:
"intelephense.files.exclude": [
"**/.git/**",
"**/.svn/**",
"**/.hg/**",
"**/CVS/**",
"**/.DS_Store/**",
"**/node_modules/**",
"**/bower_components/**",
"**/vendor/**/{Test,test,Tests,tests}/**"
],
To enable suggestions for Symfony test classes, all you need to do is edit yourĀ settings.json
Ā file and add:
"intelephense.files.exclude": [
"**/.git/**",
"**/.svn/**",
"**/.hg/**",
"**/CVS/**",
"**/.DS_Store/**",
"**/node_modules/**",
"**/bower_components/**",
"**/vendor/**/{Test,test,Tests,tests}/**/*Test.php"
],
As you can see, the last line is more specific than in the default settings.
The result š:
Code completion enabled for tests
Go to definition
When you want to get more information about a function, you can Ctrl+Click on it and VS Code will open the line where the function is declared!
Generate getters and setters for class properties
If you want VS Code to generate getters and setters for you, you can installĀ this extension. Then, right-click on a class property and selectĀ Insert PHP Getter & Setter.
Generate getter and setter
Debugging
Debugging your PHP code can be painful without a debugger. You can useĀ dump($myVar); die();
Ā (š) but itās not very convenientā¦
The best way to debug your PHP code is to useĀ Xdebug, a profiler and debugger tool for PHP.
Install Xdebug
Xdebug is not shipped with PHP, you need to install it on your development environment:
sudo apt install php-xdebug
Then runĀ sudo nano /etc/php/7.2/mods-available/xdebug.ini
Ā and add the following lines:
xdebug.remote_enable = 1
xdebug.remote_autostart = 1
Tip! If your PHP project runs in a Docker container, you need also to add the following line to the xdebug.ini
file, where 172.17.0.1
is the IP address of the docker0
interface on your computer (on Mac OS you have to put host.docker.internal
instead):
xdebug.remote_host = 172.17.0.1
Check that Xdebug is successfully installed by runningĀ php -v
. You should get something like:
PHP 7.2.19-0ubuntu0.18.04.1 (cli) (built: Jun 4 2019 14:48:12) ( NTS )
Copyright (c) 1997-2018 The PHP Group
Zend Engine v3.2.0, Copyright (c) 1998-2018 Zend Technologies
with Zend OPcache v7.2.19-0ubuntu0.18.04.1, Copyright (c) 1999-2018, by Zend Technologies
with Xdebug v2.6.0, Copyright (c) 2002-2018, by Derick Rethans
Configure VS Code debugger to listen for Xdebug
To be able to use Xdebug in VS Code, you need to install the PHP debug extension.
Then go to the debug tab in VS Code (accessible in the side menu) and click onĀ Add configuration.Ā SelectĀ PHP, and close the newly createdĀ launch.json
Ā file:
Configure VS Code debugger to listen for Xdebug
Tip! If your PHP project runs in a Docker container, you need to add the following path mapping to the launch.json
file in the Listen for Xdebug
section:
"name": "Listen for XDebug",
...
"port": 9000,
"pathMappings": {
"/path/to/app/in/docker": "${workspaceFolder}"
},
Your debugging environment is now ready! š·š
Debug your code with VS Code
Letās debug ourĀ HelloWorldController.php
Ā to see which are the query parameters given by the user. You can use the following code for example:
<?php
namespace App\Controller;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\Response;
use Symfony\Component\Routing\Annotation\Route;
class HelloWorldController
{
/**
* @Route("/hello")
*/
public function getHelloWorld(Request $request)
{
$name = $request->get('name');
return new Response('Hello '.$name.'!');
}
}
PressĀ F5Ā in VS Code to start the debugging session.
Uncheck theĀ _EverythingĀ _option in the breakpoints list and put a breakpoint at line 18 by clicking left of the line number. Then go toĀ http://127.0.0.1:8000/hello?name=Louis. You can now see the value of local variables by hovering the mouse pointer over them:
Request to http://127.0.0.1:8000/hello?name=Louis paused in debugger
You can do a lot of amazing things with the debugger š, like:
- adding or removing breakpoints
- stepping over, into or out
- watching variables
- evaluating expressions in the debug console
For more information about the power of VS Code debugger, you can visitĀ the official website.
Configure Xdebug to open file links with VS Code
When you encounter an error, or when you use the Symfony debug toolbar or the profiler, you may want to open the desired file directly in your IDE with the cursor at the corresponding line.
To be able to do that, you need to add the following line to yourĀ /etc/php/7.2/mods-available/xdebug.ini
:
xdebug.file_link_format = vscode://file/%f:%l
Tip! If you run PHP in a Docker container, you can specify a path mapping between your host and your docker like this:
xdebug.file_link_format = 'vscode://file/%f:%l&/path/to/app/in/docker/>/path/to/app/on/host/'
Now, when an error occurs, you can click on it and go directly to the corresponding line in VS Code:
When you visit a route, you can also jump to the corresponding controller by clicking on the debugging toolbar:
Formatting & linting
Formatting and linting your code is a very good practice to keep it clean automatically, and to inform you about some errors.
PHP CS Fixer
The best and commonly used linter for PHP is PHP CS Fixer. It provides a large number of rules to keep your code clean. You can visitĀ this websiteĀ for more information about the rules and the categories they belong to.
Integrate PHP CS Fixer into VS Code
To be able to auto-format your PHP files in VS Code, you need to install the php cs fixer extension.
The most complete and safe category of rules isĀ @PhpCsFixer. Itās a good point to start. To enable this set of rules in VS Code, openĀ settings.json
Ā and add the following line:
"php-cs-fixer.rules": "@PhpCsFixer",
To auto-format your PHP files on save, you also need to add the following lines to yourĀ settings.json
:
"[php]": {
"editor.defaultFormatter": "junstyle.php-cs-fixer",
"editor.formatOnSave": true
},
Auto-format on save with PHP CS Fixer
PHP CS Fixer config file
If you want to disable or enable some specific linting rules, you can do it in aĀ .php_cs
Ā file at the root folder of your project. If this configuration file is present, VS Code takes it into account and overrides the configuration found inĀ settings.json
. You can find more information about theĀ .php_cs
Ā file in theĀ GitHub repository. A simple config file to useĀ @PhpCsFixerĀ category and disable some rules could be:
<?php
return PhpCsFixer\Config::create()
->setRules([
'@PhpCsFixer' => true,
'php_unit_internal_class' => false,
'php_unit_test_class_requires_covers' => false,
])
;
Bonus: add Prettier to PHP CS Fixer
Prettier for PHPĀ is a code formatter which makes some improvements that PHP CS Fixer does not do. It allows you, among other things, to configure a max line length and makes your code even cleaner.
To add Prettier for PHP to your PHP CS Fixer configuration, you can followĀ these instructions.
In case of issues with format on save
Depending on your computerās speed, the length of your files and the number of rules you activate, linting a file on save can be slow, causing VS Code to refuse it. To fix this behavior, change theĀ formatOnSaveTimeoutĀ in yourĀ settings.json
:
"editor.formatOnSaveTimeout": 5000,
Twig support
To activate syntax highlighting for Twig files in VS Code, you need to install the Twig Language 2 extension.
To enable emmet suggestions like in HTML files, add the following line to yourĀ settings.json
:
"emmet.includeLanguages": { "twig": "html" },
Database management
A good VS Code extension to manage your databases isĀ SQLTools. Feel free to install it and manage your databases directly from your IDE!
Enjoy coding in PHP with VS Code š and feel free to give me your feedback! š
Want to learn more about how Theodo can help bring your Symfony project to the next level? Learn more about our team of Symfony experts.