From kebab-case to camelCase Using Regex: How to Refactor All Your Code in Less Than 30 seconds
Maxence Gilliot3 min read
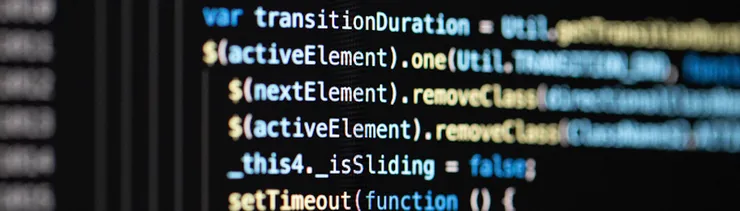
Note: This trick works on PHPStorm and Notepad++, not on VSCode.
As a coder, you must certainly have encountered a moment where you had to change multiple occurrences of a pattern. Regex are such a powerful tool for that, and this article aims at mastering them in a particular case.
The Problem
I recently worked on a VueJs project which had been using Vue.js 1.0 for 3 years. Until we decided to migrate to Vue.js 2.0. It should be quite straightforward for small projects. But ours is 50,000 lines long. Among other things, in our code, we had stuff like v-ref:my-variable
that we had to change to something like ref="myVariable"
.
Before I started crying, I wondered what could I possibly do to avoid a huge amount of refactoring.
First step: the preparation
The first step is changing v-ref:my-variable
to ref="my-variable"
. To do that, the regex is quite straightforward:
We are looking for a word in kebab-case ([\w-]+
), and we need to put it in a group (if you don’t know what a capturing group is, go here) to use it as a variable in the Replace regex: ([\w-]+)
The Find regex is v-ref:([\w-]+)
The Replace regex is ref="$1"
The $1
will be replaced by the group caught in the Find regex.
Second step: the transformation
Find regex
The second step consists of turning ref="my-variable"
to ref="myVariable"
which is equivalent to turn kebab-case to camelCase.
For that, we need to spot every occurrence of ref=
. This gives us the first part of our regex.
The second part of it is the variable name in kebab-case, which is basically a sequence of letters and possible hyphens.
Thus, given that \w
stands for a letter in regular expressions, we are looking for this pattern \w+-\w[\w-]*
, which is basically:
- a sequence of letters (
\w+
) - a hyphen (
-
) - a letter that will be capitalized (
\w
) - a sequence of letters or hyphens (
[\w-]*
)
In order to use the info from the Find regex into the Replace regex, we need to use groups, which are delimited by parentheses.
The Find regex is now ref="(\w+)-(\w)([\w-]*)"
.
Replace regex
The transformation we want to apply is:
- get rid of the hyphen;
- capitalize the first letter of the word following the hyphen.
This means we basically need to copy the first and third groups ($1
and $3
), and capitalize the second group $2
.
This is were an awesome regex trick intervenes. We are going to use the following casing operators:
\U
will set every upcoming pattern to uppercase\E
will stop any casing transformation (such as\U
)
The Replace regex is thus ref="$1\U$2\E$3"
.
Warning
We assumed there was only one hyphen in the variable name. In case there are more than one, we could simply re-apply the Find and replace of the second step until no occurrence is found.
Summary
My problem is I want to go from v-ref:my-awesome-variable
to ref="myAwesomeVariable"
First step
The Find regex is v-ref:([\w-]+)
The Replace regex is thus ref="$1"
Second step (to iterate several times)
The Find regex is ref="(\w+)-(\w)([\w-]*)"
The Replace regex is thus ref="$1\U$2\E$3"
The trick is to apply the second step as long as some occurrences of the pattern are found.
Thus, a variable like my-awesome-variable
would have been transformed as follows:
v-ref:my-awesome-variable
→ref="my-awesome-variable"
(first step)ref="my-awesome-variable"
→ref="myAwesome-variable"
(second step)ref="myAwesome-variable"
→ref="myAwesomeVariable"
(second step again)