Making Amazon Dash Buttons Useful: By building a doorbell!
Ben Ellerby3 min read
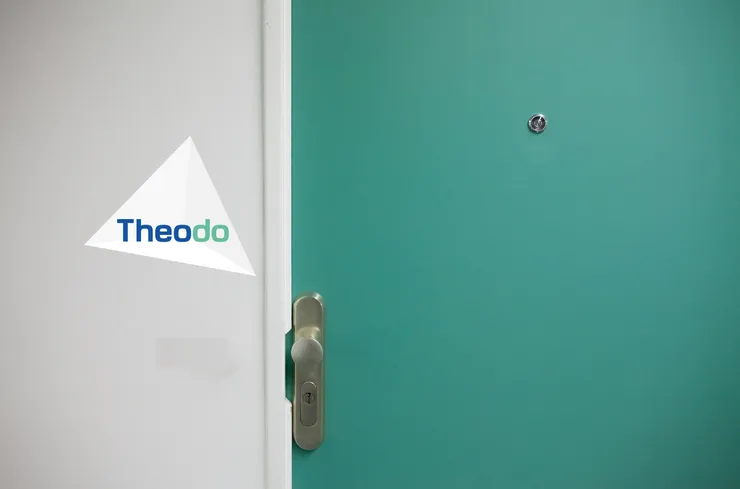
A few years ago Amazon came out with the Amazon Dash Button, a small internet connected button that can be used to reorder common household items. Such a small, cheap and well-made internet connected button seems like a godsend for the IOT developer community - but they are not intended for use outside of product ordering. Amazon did release an IOT version of the Dash button, but at 4 times the price point it’s less attractive.
Could we intercept the network requests from Dash buttons and trigger our own custom events? An IOT doorbell, office coffee emergency button… That’s the challenge we set ourselves.
Connect the Dash Button to WiFi
Open up your Amazon app on IOS or Android, turn on Bluetooth and add the device. Be sure to quit the process once it’s connected to the internet but before you select a specific product!
Now your button is online.
Getting the MAC address of the button
The Dash Button is asleep most of the time, meaning when you press the button it must connect to the LAN over WiFi and then send its API request. Therefore it needs to acquire an IP address.
On an IPv4 network, Dynamic Host Configuration Protocol (DHCP) is used to get an address, and this process includes an Address Resolution Protocol (ARP) request. Our plan is to place a Raspberry Pi device on the LAN to watch for such ARP requests from our Dash buttons, allowing us to then trigger actions.
From our laptop on the same network, we can watch broadcast packets on the network using tcpdump.To watch for ARP request packets we can run:
[code languare=“bash”]
tcpdump -ve arp | awk ‘{print $2}
(on Mac)
- the -v option gives us a verbose output
- the -e option displays the MAC addresses
- the awk script prints out just the MAC address column.
There will likely be many busy devices cluttering up your network so run this, press the dash button, wait 30 seconds and then look at the list of MAC addresses captured.
Part of the MAC address specification includes the manufacturers ID so we can go to an online tool such as macvendors.com and lookup each of the addresses we found. The one that has the manufacturer as Amazon Technologies Inc. is what you’re looking for. Make a note of the address in question, and if you’re looking to setup multiple devices use the same process.
If you have other Amazon devices on your network this process may take more trial and error.
Triggering an action
We can write a short bash script, using a similar approach to above, that will trigger an action whenever the button is pressed.
#!/bin/bash
button='ENTER_THE_MAC_ADDRESS'
tcpdump -l -n -i en0 | while read b; do
if echo $b | grep -q $button ; then
echo "Trigger Action"
fi
done
- button is the MAC address from the step above
- tcpdump is passed an interface using the -i option. en0 or eth0 will likely work for you, but use ifconfig to find you LAN ethernet interface.
- We’re piping into a while loop to get around some issues with evaluating our post grep action.
We can now trigger any action based on the pressing of the button. This could be an SMS using Twilio, an email, voice announcement using espeak, an AWS Lambda function… the world’s your limit!
Making a doorbell
If we want our doorbell to drop us an SMS we can sign up for Twilio’s programmable SMS service.
Then we can adapt our script to include a curl request as follows:
#!/bin/bash
button='ENTER_THE_MAC_ADDRESS'