Create a PHP AI agent with LLPhant (powered by OpenAI)
Thomas Hercule8 min read
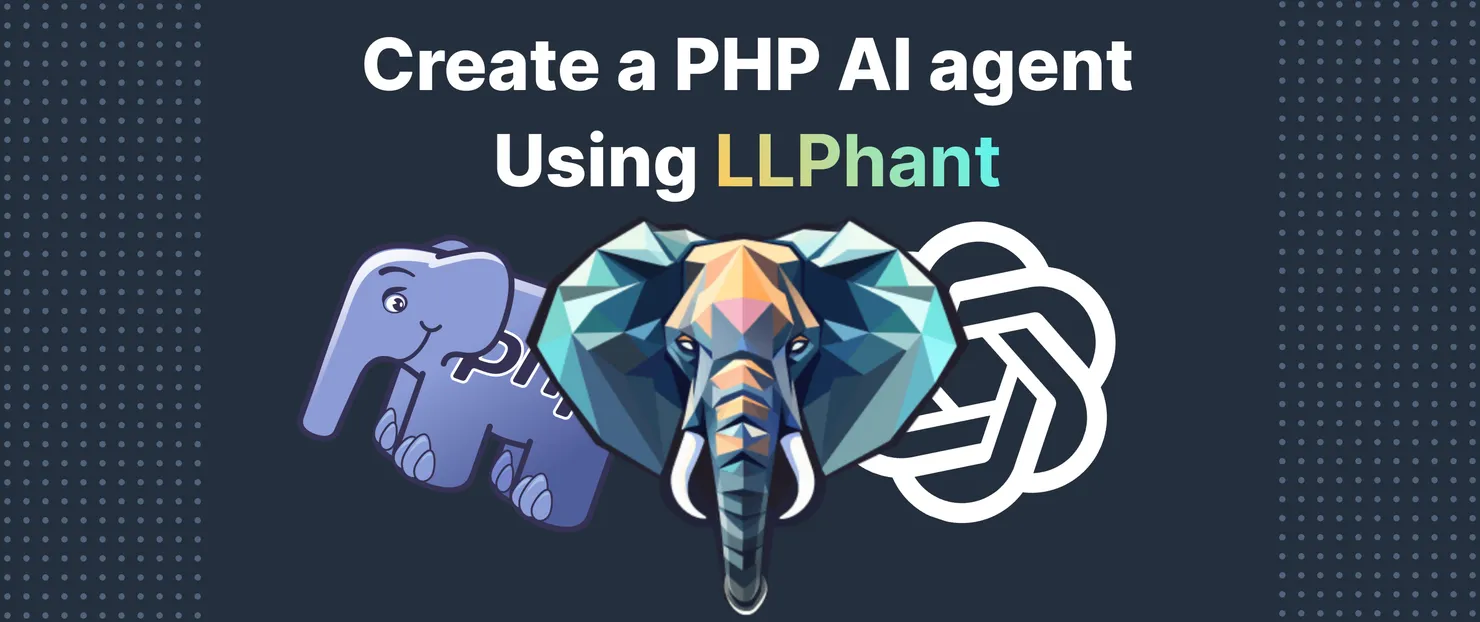
Automation is the lifeblood of modern tech. Whether it is managing e-commerce websites, providing customer support, optimizing sales, or complex searches, the power of automation can not be overstated. Let’s explore how it can transform complex tasks with the magic of OpenAI on your PHP project.
What tool to use to automate PHP tasks with OpenAI
AutoPHP is a tool from the open-source PHP generative AI framework LLphant 🐘 (by Theodo). AutoPHP fine-tune GPT engine to generate high-quality results from a given objective. To do so, it allows GPT to create tasks for himself and use PHP functions. AutoPHP is greatly inspired by AutoGPT but for PHP applications. While it is still in development and not as good as AutoGPT at the moment, it still shows great potential and can be used right now for simple tasks.
How does a PHP AI agent work?
AutoPHP uses GPT, so it takes sentences as input, more precisely as an objective.
AutoPHP simple Example
GPT 3.5 Turbo is used for this example.
Currently, AutoPHP is working well for simple tasks that require some PHP functions. For example, if we want to find the name and age of the French player who scored the most tries during the 2023 Rugby World Cup.
Prerequisites : LLPhant composer require theodo-group/llphant
First, import the libraries:
<?php
use LLPhant\Experimental\Agent\AutoPHP;
require_once 'vendor/autoload.php';
Then describe the objective
$objective = 'Find the name and age of the french player who scored the most tries during the 2023 rugby world cup.';
Add some PHP functions to the agent so it can use them
In this example, we are going to add SerpApiSearch which is a tool from the LLPhant framework that performs a Google search using the SerpApi and returns the title and snippet of the first results of the Google search.
$searchApi = new SerpApiSearch('VERY_REAL_API_KEY');
$searchFunction = FunctionBuilder::buildFunctionInfo($searchApi, 'googleSearch');
Finally, create an AutoPHP instance with the objective and the available functions, and run it!
// use LLPhant\Experimental\Agent\AutoPHP
$AutoPHP = new AutoPHP($objective, [$searchFunction]);
$response = $AutoPHP->run();
AutoPHP’s response
First, he defines a list of tasks:
Then he tries to do the first one (here using the SerpApi):
It finds the player and updates the list of tasks:
It made one more call to the SerpApi, which was enough to get the age directly, so it finished.
Now let’s try with a more complex example.
AutoPHP complex Example
Let’s put AutoPHP to the test with a harder example (GPT 4 engine is used).
Scenario: Automating Content Aggregation for a News Website
Imagine you’re running a news website about generative AI that aims to provide readers with up-to-the-minute news stories from a diverse range of sources. The challenge is not only to gather articles but also to categorize them, summarize their content, and translate them into English if needed. All of this needs to be done efficiently and consistently.
Building the AutoPHP Function
To achieve this, we’re going to use AutoPHP. We’ll define a specific objective with some instructions to help him. The objective :
You have to recover and process data for a news website focused on generative AI. Select FIVE articles url about generative AI using google. Then you HAVE TO get the content of the web page in order to create a summary the article (if you can t get the content from the url, you can use the meta description). Then categorize the article by topics such as research, applications, and breakthroughs. Give a formatted response with : title, url, summary, topics.
We will also provide him with the tools he needs to complete the task :
- “WebPageTextGetter” to get the content of the web pages.
- ”SerpApiSearch” to search for articles about generative AI using the SerpApi.
<?php
use LLPhant\Chat\FunctionInfo\FunctionBuilder;
use LLPhant\Experimental\Agent\AutoPHP;
use LLPhant\Tool\SerpApiSearch;
use LLPhant\Tool\WebPageTextGetter;
require_once 'vendor/autoload.php';
$objective = '
You have to recover and process data for a news website focused on generative AI. Select FIVE articles url about generative AI using google. Then you HAVE TO get the content of the web page in order to create a summary the article (if you can t get the content from the url, you can use the meta description). Then categorize the article by topics such as research, applications, and breakthroughs. Give a formatted response with : title, url, summary, topics.
';
$verbose = true;
$getWebPageText = new WebPageTextGetter($verbose);
$getMultipleWebPageText = FunctionBuilder::buildFunctionInfo($getWebPageText, 'getMultipleWebPageText');
$searchApi = new SerpApiSearch('VERY_REAL_API_KEY', $verbose);
$search = FunctionBuilder::buildFunctionInfo($searchApi, 'googleSearch');
$AutoPHP = new AutoPHP($objective, [$getMultipleWebPageText, $search], $verbose);
$response = $AutoPHP->run();
AutoPHP Execution
As always, AutoPHP starts by defining a list of tasks:
It searches for articles about generative AI using the SerpApi, then it gets the content of the web pages using the “WebPageTextGetter” tool :
Finnaly, it returns the result:
Unfortunately, all not articles have the required informations. All the articles should have a URL, a title, a summary and topics.
- 🟢 Article 2 have everything.
- 🟠 Article 1 and 3 lacks URL.
- 🔴 Article 4 and 5 lacks pretty much everything.
AutoPHP is in active development, ensuring it will handle this task within a few months, possibly sooner if you’re interested in contributing to LLPhant! Don’t hesitate to reach out; I’d be delighted to assist with your first contribution.
Conclusion: The Future of Task Automation with AutoPHP
In conclusion, AutoPHP will soon be a game-changing tool that empowers your tech startup with task automation, powered by OpenAI.
AutoPHP streamlines complex tasks, making them more accessible and efficient. It is time to explore the capabilities of AutoPHP and embrace the future of task automation.